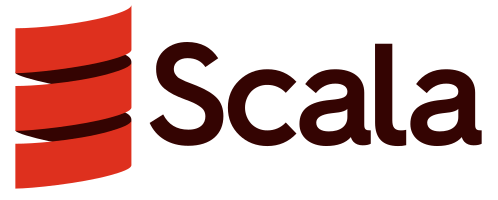
Scala (/ˈskɑːlɑː/ SKAH-lah)[7][8] is a strong statically typed high-level general-purpose programming language that supports both object-oriented programming and functional programming. Designed to be concise,[9] many of Scala's design decisions are intended to address criticisms of Java.[6]
Scala source code can be compiled to Java bytecode and run on a Java virtual machine (JVM). Scala can also be transpiled to JavaScript to run in a browser, or compiled directly to a native executable. When running on the JVM, Scala provides language interoperability with Java so that libraries written in either language may be referenced directly in Scala or Java code.[10] Like Java, Scala is object-oriented, and uses a syntax termed curly-brace which is similar to the language C. Since Scala 3, there is also an option to use the off-side rule (indenting) to structure blocks, and its use is advised. Martin Odersky has said that this turned out to be the most productive change introduced in Scala 3.[11]
Unlike Java, Scala has many features of functional programming languages (like Scheme, Standard ML, and Haskell), including currying, immutability, lazy evaluation, and pattern matching. It also has an advanced type system supporting algebraic data types, covariance and contravariance, higher-order types (but not higher-rank types), anonymous types, operator overloading, optional parameters, named parameters, raw strings, and an experimental exception-only version of algebraic effects that can be seen as a more powerful version of Java's checked exceptions.[12]
The name Scala is a portmanteau of scalable and language, signifying that it is designed to grow with the demands of its users.[13]
History
The design of Scala started in 2001 at the École Polytechnique Fédérale de Lausanne (EPFL) (in Lausanne, Switzerland) by Martin Odersky. It followed on from work on Funnel, a programming language combining ideas from functional programming and Petri nets.[14] Odersky formerly worked on Generic Java, and javac, Sun's Java compiler.[14]
After an internal release in late 2003, Scala was released publicly in early 2004 on the Java platform,[15][6][14][16] A second version (v2.0) followed in March 2006.[6]
On 17 January 2011, the Scala team won a five-year research grant of over €2.3 million from the European Research Council.[17] On 12 May 2011, Odersky and collaborators launched Typesafe Inc. (later renamed Lightbend Inc.), a company to provide commercial support, training, and services for Scala. Typesafe received a $3 million investment in 2011 from Greylock Partners.[18][19][20][21]
As of June 2024, Scala maintainers are planning on delivering version 3.6 by the end of 2024. They are concentrating on improving a number of features, such as decreasing build times, achieving closer integration with tooling, enriching user experience (UX), improving reporting, and refining the compiler’s stability and accuracy.[22]
Platforms and license
Scala runs on the Java platform (Java virtual machine) and is compatible with existing Java programs.[15] As Android applications are typically written in Java and translated from Java bytecode into Dalvik bytecode (which may be further translated to native machine code during installation) when packaged, Scala's Java compatibility makes it well-suited to Android development, the more so when a functional approach is preferred.[23]
The reference Scala software distribution, including compiler and libraries, is released under the Apache license.[24]
Other compilers and targets
Scala.js is a Scala compiler that compiles to JavaScript, making it possible to write Scala programs that can run in web browsers or Node.js.[25] The compiler, in development since 2013, was announced as no longer experimental in 2015 (v0.6). Version v1.0.0-M1 was released in June 2018 and version 1.1.1 in September 2020.[26]
Scala Native is a Scala compiler that targets the LLVM compiler infrastructure to create executable code that uses a lightweight managed runtime, which uses the Boehm garbage collector. The project is led by Denys Shabalin and had its first release, 0.1, on 14 March 2017. Development of Scala Native began in 2015 with a goal of being faster than just-in-time compilation for the JVM by eliminating the initial runtime compilation of code and also providing the ability to call native routines directly.[27][28]
A reference Scala compiler targeting the .NET Framework and its Common Language Runtime was released in June 2004,[14] but was officially dropped in 2012.[29]
Examples
"Hello World" example
The Hello World program written in Scala 3 has this form:
@main def main() = println("Hello, World!")
Unlike the stand-alone Hello World application for Java, there is no class declaration and nothing is declared to be static.
When the program is stored in file HelloWorld.scala, the user compiles it with the command:
$ scalac HelloWorld.scala
and runs it with
$ scala HelloWorld
This is analogous to the process for compiling and running Java code. Indeed, Scala's compiling and executing model is identical to that of Java, making it compatible with Java build tools such as Apache Ant.
A shorter version of the "Hello World" Scala program is:
println("Hello, World!")
Scala includes an interactive shell and scripting support.[30] Saved in a file named HelloWorld2.scala
, this can be run as a script using the command:
$ scala HelloWorld2.scala
Commands can also be entered directly into the Scala interpreter, using the option -e:
$ scala -e 'println("Hello, World!")'
Expressions can be entered interactively in the REPL:
$ scala
Welcome to Scala 2.12.2 (Java HotSpot(TM) 64-Bit Server VM, Java 1.8.0_131).
Type in expressions for evaluation. Or try :help.
scala> List(1, 2, 3).map(x => x * x)
res0: List[Int] = List(1, 4, 9)
scala>
Basic example
The following example shows the differences between Java and Scala syntax. The function mathFunction takes an integer, squares it, and then adds the cube root of that number to the natural log of that number, returning the result (i.e., ):
Some syntactic differences in this code are:
- Scala does not require semicolons (;) to end statements.
- Value types are capitalized (sentence case):
Int, Double, Boolean
instead ofint, double, boolean
. - Parameter and return types follow, as in Pascal, rather than precede as in C.
- Methods must be preceded by
def
. - Local or class variables must be preceded by
val
(indicates an immutable variable) orvar
(indicates a mutable variable). - The
return
operator is unnecessary in a function (although allowed); the value of the last executed statement or expression is normally the function's value. - Instead of the Java cast operator
(Type) foo
, Scala usesfoo.asInstanceOf[Type]
, or a specialized function such astoDouble
ortoInt
. - Instead of Java's
import foo.*;
, Scala usesimport foo._
. - Function or method
foo()
can also be called as justfoo
; methodthread.send(signo)
can also be called as justthread send signo
; and methodfoo.toString()
can also be called as justfoo toString
.
These syntactic relaxations are designed to allow support for domain-specific languages.
Some other basic syntactic differences:
- Array references are written like function calls, e.g.
array(i)
rather thanarray[i]
. (Internally in Scala, the former expands into array.apply(i) which returns the reference) - Generic types are written as e.g.
List[String]
rather than Java'sList<String>
. - Instead of the pseudo-type
void
, Scala has the actual singleton classUnit
(see below).
Example with classes
The following example contrasts the definition of classes in Java and Scala.
The code above shows some of the conceptual differences between Java and Scala's handling of classes:
- Scala has no static variables or methods. Instead, it has singleton objects, which are essentially classes with only one instance. Singleton objects are declared using
object
instead ofclass
. It is common to place static variables and methods in a singleton object with the same name as the class name, which is then known as a companion object.[15] (The underlying class for the singleton object has a$
appended. Hence, forclass Foo
with companion objectobject Foo
, under the hood there's a classFoo$
containing the companion object's code, and one object of this class is created, using the singleton pattern.) - In place of constructor parameters, Scala has class parameters, which are placed on the class, similar to parameters to a function. When declared with a
val
orvar
modifier, fields are also defined with the same name, and automatically initialized from the class parameters. (Under the hood, external access to public fields always goes through accessor (getter) and mutator (setter) methods, which are automatically created. The accessor function has the same name as the field, which is why it's unnecessary in the above example to explicitly declare accessor methods.) Note that alternative constructors can also be declared, as in Java. Code that would go into the default constructor (other than initializing the member variables) goes directly at class level. - In Scala it is possible to define operators by using symbols as method names. In place of
addPoint
, the Scala example defines+=
, which is then invoked with infix notation asgrid += this
. - Default visibility in Scala is
public
.
Features (with reference to Java)
Scala has the same compiling model as Java and C#, namely separate compiling and dynamic class loading, so that Scala code can call Java libraries.
Scala's operational characteristics are the same as Java's. The Scala compiler generates byte code that is nearly identical to that generated by the Java compiler.[15] In fact, Scala code can be decompiled to readable Java code, with the exception of certain constructor operations. To the Java virtual machine (JVM), Scala code and Java code are indistinguishable. The only difference is one extra runtime library, scala-library.jar
.[31]
Scala adds a large number of features compared with Java, and has some fundamental differences in its underlying model of expressions and types, which make the language theoretically cleaner and eliminate several corner cases in Java. From the Scala perspective, this is practically important because several added features in Scala are also available in C#.
Syntactic flexibility
As mentioned above, Scala has a good deal of syntactic flexibility, compared with Java. The following are some examples:
- Semicolons are unnecessary; lines are automatically joined if they begin or end with a token that cannot normally come in this position, or if there are unclosed parentheses or brackets.
- Any method can be used as an infix operator, e.g.
"%d apples".format(num)
and"%d apples" format num
are equivalent. In fact, arithmetic operators like+
and<<
are treated just like any other methods, since function names are allowed to consist of sequences of arbitrary symbols (with a few exceptions made for things like parens, brackets and braces that must be handled specially); the only special treatment that such symbol-named methods undergo concerns the handling of precedence. - Methods
apply
andupdate
have syntactic short forms.foo()
—wherefoo
is a value (singleton object or class instance)—is short forfoo.apply()
, andfoo() = 42
is short forfoo.update(42)
. Similarly,foo(42)
is short forfoo.apply(42)
, andfoo(4) = 2
is short forfoo.update(4, 2)
. This is used for collection classes and extends to many other cases, such as STM cells. - Scala distinguishes between no-parens (
def foo = 42
) and empty-parens (def foo() = 42
) methods. When calling an empty-parens method, the parentheses may be omitted, which is useful when calling into Java libraries that do not know this distinction, e.g., usingfoo.toString
instead offoo.toString()
. By convention, a method should be defined with empty-parens when it performs side effects. - Method names ending in colon (
:
) expect the argument on the left-hand-side and the receiver on the right-hand-side. For example, the4 :: 2 :: Nil
is the same asNil.::(2).::(4)
, the first form corresponding visually to the result (a list with first element 4 and second element 2). - Class body variables can be transparently implemented as separate getter and setter methods. For
trait FooLike { var bar: Int }
, an implementation may beobject Foo extends FooLike { private var x = 0; def bar = x; def bar_=(value: Int) { x = value }} } }
. The call site will still be able to use a concisefoo.bar = 42
. - The use of curly braces instead of parentheses is allowed in method calls. This allows pure library implementations of new control structures.[32] For example,
breakable { ... if (...) break() ... }
looks as ifbreakable
was a language defined keyword, but really is just a method taking a thunk argument. Methods that take thunks or functions often place these in a second parameter list, allowing to mix parentheses and curly braces syntax:Vector.fill(4) { math.random }
is the same asVector.fill(4)(math.random)
. The curly braces variant allows the expression to span multiple lines. - For-expressions (explained further down) can accommodate any type that defines monadic methods such as
map
,flatMap
andfilter
.
By themselves, these may seem like questionable choices, but collectively they serve the purpose of allowing domain-specific languages to be defined in Scala without needing to extend the compiler. For example, Erlang's special syntax for sending a message to an actor, i.e. actor ! message
can be (and is) implemented in a Scala library without needing language extensions.
Unified type system
Java makes a sharp distinction between primitive types (e.g. int
and boolean
) and reference types (any class). Only reference types are part of the inheritance scheme, deriving from java.lang.Object
. In Scala, all types inherit from a top-level class Any
, whose immediate children are AnyVal
(value types, such as Int
and Boolean
) and AnyRef
(reference types, as in Java). This means that the Java distinction between primitive types and boxed types (e.g. int
vs. Integer
) is not present in Scala; boxing and unboxing is completely transparent to the user. Scala 2.10 allows for new value types to be defined by the user.
For-expressions
Instead of the Java "foreach" loops for looping through an iterator, Scala has for
-expressions, which are similar to list comprehensions in languages such as Haskell, or a combination of list comprehensions and generator expressions in Python. For-expressions using the yield
keyword allow a new collection to be generated by iterating over an existing one, returning a new collection of the same type. They are translated by the compiler into a series of map
, flatMap
and filter
calls. Where yield
is not used, the code approximates to an imperative-style loop, by translating to foreach
.
A simple example is:
val s = for (x <- 1 to 25 if x*x > 50) yield 2*x
The result of running it is the following vector:
Vector(16, 18, 20, 22, 24, 26, 28, 30, 32, 34, 36, 38, 40, 42, 44, 46, 48, 50)
(Note that the expression 1 to 25
is not special syntax. The method to
is rather defined in the standard Scala library as an extension method on integers, using a technique known as implicit conversions[33] that allows new methods to be added to existing types.)
A more complex example of iterating over a map is:
// Given a map specifying Twitter users mentioned in a set of tweets,
// and number of times each user was mentioned, look up the users
// in a map of known politicians, and return a new map giving only the
// Democratic politicians (as objects, rather than strings).
val dem_mentions = for
(mention, times) <- mentions
account <- accounts.get(mention)
if account.party == "Democratic"
yield (account, times)
Expression (mention, times) <- mentions
is an example of pattern matching (see below). Iterating over a map returns a set of key-value tuples, and pattern-matching allows the tuples to easily be destructured into separate variables for the key and value. Similarly, the result of the comprehension also returns key-value tuples, which are automatically built back up into a map because the source object (from the variable mentions
) is a map. Note that if mentions
instead held a list, set, array or other collection of tuples, exactly the same code above would yield a new collection of the same type.
Functional tendencies
While supporting all of the object-oriented features available in Java (and in fact, augmenting them in various ways), Scala also provides a large number of capabilities that are normally found only in functional programming languages. Together, these features allow Scala programs to be written in an almost completely functional style and also allow functional and object-oriented styles to be mixed.
Examples are:
- No distinction between statements and expressions
- Type inference
- Anonymous functions with capturing semantics (i.e., closures)
- Immutable variables and objects
- Lazy evaluation
- Delimited continuations (since 2.8)
- Higher-order functions
- Nested functions
- Currying
- Pattern matching
- Algebraic data types (through case classes)
- Tuples
Everything is an expression
Unlike C or Java, but similar to languages such as Lisp, Scala makes no distinction between statements and expressions. All statements are in fact expressions that evaluate to some value. Functions that would be declared as returning void
in C or Java, and statements like while
that logically do not return a value, are in Scala considered to return the type Unit
, which is a singleton type, with only one object of that type. Functions and operators that never return at all (e.g. the throw
operator or a function that always exits non-locally using an exception) logically have return type Nothing
, a special type containing no objects; that is, a bottom type, i.e. a subclass of every possible type. (This in turn makes type Nothing
compatible with every type, allowing type inference to function correctly.)[34]
Similarly, an if-then-else
"statement" is actually an expression, which produces a value, i.e. the result of evaluating one of the two branches. This means that such a block of code can be inserted wherever an expression is desired, obviating the need for a ternary operator in Scala:
For similar reasons, return
statements are unnecessary in Scala, and in fact are discouraged. As in Lisp, the last expression in a block of code is the value of that block of code, and if the block of code is the body of a function, it will be returned by the function.
To make it clear that all functions are expressions, even methods that return Unit
are written with an equals sign
def printValue(x: String): Unit =
println("I ate a %s".format(x))
or equivalently (with type inference, and omitting the unnecessary newline):
def printValue(x: String) = println("I ate a %s" format x)
Type inference
Due to type inference, the type of variables, function return values, and many other expressions can typically be omitted, as the compiler can deduce it. Examples are val x = "foo"
(for an immutable constant or immutable object) or var x = 1.5
(for a variable whose value can later be changed). Type inference in Scala is essentially local, in contrast to the more global Hindley-Milner algorithm used in Haskell, ML and other more purely functional languages. This is done to facilitate object-oriented programming. The result is that certain types still need to be declared (most notably, function parameters, and the return types of recursive functions), e.g.
def formatApples(x: Int) = "I ate %d apples".format(x)
or (with a return type declared for a recursive function)
def factorial(x: Int): Int =
if x == 0 then 1
else x * factorial(x - 1)
Anonymous functions
In Scala, functions are objects, and a convenient syntax exists for specifying anonymous functions. An example is the expression x => x < 2
, which specifies a function with one parameter, that compares its argument to see if it is less than 2. It is equivalent to the Lisp form (lambda (x) (< x 2))
. Note that neither the type of x
nor the return type need be explicitly specified, and can generally be inferred by type inference; but they can be explicitly specified, e.g. as (x: Int) => x < 2
or even (x: Int) => (x < 2): Boolean
.
Anonymous functions behave as true closures in that they automatically capture any variables that are lexically available in the environment of the enclosing function. Those variables will be available even after the enclosing function returns, and unlike in the case of Java's anonymous inner classes do not need to be declared as final. (It is even possible to modify such variables if they are mutable, and the modified value will be available the next time the anonymous function is called.)
An even shorter form of anonymous function uses placeholder variables: For example, the following:
list map { x => sqrt(x) }
can be written more concisely as
list map { sqrt(_) }
or even
list map sqrt
Immutability
Scala enforces a distinction between immutable and mutable variables. Mutable variables are declared using the var
keyword and immutable values are declared using the val
keyword.
A variable declared using the val
keyword cannot be reassigned in the same way that a variable declared using the final
keyword can't be reassigned in Java. val
s are only shallowly immutable, that is, an object referenced by a val is not guaranteed to itself be immutable.
Immutable classes are encouraged by convention however, and the Scala standard library provides a rich set of immutable collection classes. Scala provides mutable and immutable variants of most collection classes, and the immutable version is always used unless the mutable version is explicitly imported.[35] The immutable variants are persistent data structures that always return an updated copy of an old object instead of updating the old object destructively in place. An example of this is immutable linked lists where prepending an element to a list is done by returning a new list node consisting of the element and a reference to the list tail. Appending an element to a list can only be done by prepending all elements in the old list to a new list with only the new element. In the same way, inserting an element in the middle of a list will copy the first half of the list, but keep a reference to the second half of the list. This is called structural sharing. This allows for very easy concurrency — no locks are needed as no shared objects are ever modified.[36]
Lazy (non-strict) evaluation
Evaluation is strict ("eager") by default. In other words, Scala evaluates expressions as soon as they are available, rather than as needed. However, it is possible to declare a variable non-strict ("lazy") with the lazy
keyword, meaning that the code to produce the variable's value will not be evaluated until the first time the variable is referenced. Non-strict collections of various types also exist (such as the type Stream
, a non-strict linked list), and any collection can be made non-strict with the view
method. Non-strict collections provide a good semantic fit to things like server-produced data, where the evaluation of the code to generate later elements of a list (that in turn triggers a request to a server, possibly located somewhere else on the web) only happens when the elements are actually needed.
Tail recursion
Functional programming languages commonly provide tail call optimization to allow for extensive use of recursion without stack overflow problems. Limitations in Java bytecode complicate tail call optimization on the JVM. In general, a function that calls itself with a tail call can be optimized, but mutually recursive functions cannot. Trampolines have been suggested as a workaround.[37] Trampoline support has been provided by the Scala library with the object scala.util.control.TailCalls
since Scala 2.8.0 (released 14 July 2010). A function may optionally be annotated with @tailrec
, in which case it will not compile unless it is tail recursive.[38]
An example of this optimization could be implemented using the factorial definition. For instance, the recursive version of the factorial:
def factorial(n: Int): Int =
if n == 0 then 1
else n * factorial(n - 1)
Could be optimized to the tail recursive version like this:
@tailrec
def factorial(n: Int, accum: Int): Int =
if n == 0 then accum
else factorial(n - 1, n * accum)
However, this could compromise composability with other functions because of the new argument on its definition, so it is common to use closures to preserve its original signature:
def factorial(n: Int): Int =
@tailrec
def loop(current: Int, accum: Int): Int =
if n == 0 then accum
else loop(current - 1, n * accum)
loop(n, 1) // Call to the closure using the base case
end factorial
This ensures tail call optimization and thus prevents a stack overflow error.
Case classes and pattern matching
Scala has built-in support for pattern matching, which can be thought of as a more sophisticated, extensible version of a switch statement, where arbitrary data types can be matched (rather than just simple types like integers, Booleans and strings), including arbitrary nesting. A special type of class known as a case class is provided, which includes automatic support for pattern matching and can be used to model the algebraic data types used in many functional programming languages. (From the perspective of Scala, a case class is simply a normal class for which the compiler automatically adds certain behaviors that could also be provided manually, e.g., definitions of methods providing for deep comparisons and hashing, and destructuring a case class on its constructor parameters during pattern matching.)
An example of a definition of the quicksort algorithm using pattern matching is this:
def qsort(list: List[Int]): List[Int] = list match
case Nil => Nil
case pivot :: tail =>
val (smaller, rest) = tail.partition(_ < pivot)
qsort(smaller) ::: pivot :: qsort(rest)
The idea here is that we partition a list into the elements less than a pivot and the elements not less, recursively sort each part, and paste the results together with the pivot in between. This uses the same divide-and-conquer strategy of mergesort and other fast sorting algorithms.
The match
operator is used to do pattern matching on the object stored in list
. Each case
expression is tried in turn to see if it will match, and the first match determines the result. In this case, Nil
only matches the literal object Nil
, but pivot :: tail
matches a non-empty list, and simultaneously destructures the list according to the pattern given. In this case, the associated code will have access to a local variable named pivot
holding the head of the list, and another variable tail
holding the tail of the list. Note that these variables are read-only, and are semantically very similar to variable bindings established using the let
operator in Lisp and Scheme.
Pattern matching also happens in local variable declarations. In this case, the return value of the call to tail.partition
is a tuple — in this case, two lists. (Tuples differ from other types of containers, e.g. lists, in that they are always of fixed size and the elements can be of differing types — although here they are both the same.) Pattern matching is the easiest way of fetching the two parts of the tuple.
The form _ < pivot
is a declaration of an anonymous function with a placeholder variable; see the section above on anonymous functions.
The list operators ::
(which adds an element onto the beginning of a list, similar to cons
in Lisp and Scheme) and :::
(which appends two lists together, similar to append
in Lisp and Scheme) both appear. Despite appearances, there is nothing "built-in" about either of these operators. As specified above, any string of symbols can serve as function name, and a method applied to an object can be written "infix"-style without the period or parentheses. The line above as written:
qsort(smaller) ::: pivot :: qsort(rest)
could also be written thus:
qsort(rest).::(pivot).:::(qsort(smaller))
in more standard method-call notation. (Methods that end with a colon are right-associative and bind to the object to the right.)
Partial functions
In the pattern-matching example above, the body of the match
operator is a partial function, which consists of a series of case
expressions, with the first matching expression prevailing, similar to the body of a switch statement. Partial functions are also used in the exception-handling portion of a try
statement:
try
...
catch
case nfe: NumberFormatException => { println(nfe); List(0) }
case _ => Nil
Finally, a partial function can be used alone, and the result of calling it is equivalent to doing a match
over it. For example, the prior code for quicksort can be written thus:
val qsort: List[Int] => List[Int] =
case Nil => Nil
case pivot :: tail =>
val (smaller, rest) = tail.partition(_ < pivot)
qsort(smaller) ::: pivot :: qsort(rest)
Here a read-only variable is declared whose type is a function from lists of integers to lists of integers, and bind it to a partial function. (Note that the single parameter of the partial function is never explicitly declared or named.) However, we can still call this variable exactly as if it were a normal function:
scala> qsort(List(6,2,5,9))
res32: List[Int] = List(2, 5, 6, 9)
Object-oriented extensions
Scala is a pure object-oriented language in the sense that every value is an object. Data types and behaviors of objects are described by classes and traits. Class abstractions are extended by subclassing and by a flexible mixin-based composition mechanism to avoid the problems of multiple inheritance.
Traits are Scala's replacement for Java's interfaces. Interfaces in Java versions under 8 are highly restricted, able only to contain abstract function declarations. This has led to criticism that providing convenience methods in interfaces is awkward (the same methods must be reimplemented in every implementation), and extending a published interface in a backwards-compatible way is impossible. Traits are similar to mixin classes in that they have nearly all the power of a regular abstract class, lacking only class parameters (Scala's equivalent to Java's constructor parameters), since traits are always mixed in with a class. The super
operator behaves specially in traits, allowing traits to be chained using composition in addition to inheritance. The following example is a simple window system:
abstract class Window:
// abstract
def draw()
class SimpleWindow extends Window:
def draw()
println("in SimpleWindow")
// draw a basic window
trait WindowDecoration extends Window
trait HorizontalScrollbarDecoration extends WindowDecoration:
// "abstract override" is needed here for "super()" to work because the parent
// function is abstract. If it were concrete, regular "override" would be enough.
abstract override def draw()
println("in HorizontalScrollbarDecoration")
super.draw()
// now draw a horizontal scrollbar
trait VerticalScrollbarDecoration extends WindowDecoration:
abstract override def draw()
println("in VerticalScrollbarDecoration")
super.draw()
// now draw a vertical scrollbar
trait TitleDecoration extends WindowDecoration:
abstract override def draw()
println("in TitleDecoration")
super.draw()
// now draw the title bar
A variable may be declared thus:
val mywin = new SimpleWindow with VerticalScrollbarDecoration with HorizontalScrollbarDecoration with TitleDecoration
The result of calling mywin.draw()
is:
in TitleDecoration
in HorizontalScrollbarDecoration
in VerticalScrollbarDecoration
in SimpleWindow
In other words, the call to draw
first executed the code in TitleDecoration
(the last trait mixed in), then (through the super()
calls) threaded back through the other mixed-in traits and eventually to the code in Window
, even though none of the traits inherited from one another. This is similar to the decorator pattern, but is more concise and less error-prone, as it doesn't require explicitly encapsulating the parent window, explicitly forwarding functions whose implementation isn't changed, or relying on run-time initialization of entity relationships. In other languages, a similar effect could be achieved at compile-time with a long linear chain of implementation inheritance, but with the disadvantage compared to Scala that one linear inheritance chain would have to be declared for each possible combination of the mix-ins.
Expressive type system
Scala is equipped with an expressive static type system that mostly enforces the safe and coherent use of abstractions. The type system is, however, not sound.[39] In particular, the type system supports:
- Classes and abstract types as object members
- Structural types
- Path-dependent types
- Compound types
- Explicitly typed self references
- Generic classes
- Polymorphic methods
- Upper and lower type bounds
- Variance
- Annotation
- Views
Scala is able to infer types by use. This makes most static type declarations optional. Static types need not be explicitly declared unless a compiler error indicates the need. In practice, some static type declarations are included for the sake of code clarity.
Type enrichment
A common technique in Scala, known as "enrich my library"[40] (originally termed "pimp my library" by Martin Odersky in 2006;[33] concerns were raised about this phrasing due to its negative connotations[41] and immaturity[42]), allows new methods to be used as if they were added to existing types. This is similar to the C# concept of extension methods but more powerful, because the technique is not limited to adding methods and can, for instance, be used to implement new interfaces. In Scala, this technique involves declaring an implicit conversion from the type "receiving" the method to a new type (typically, a class) that wraps the original type and provides the additional method. If a method cannot be found for a given type, the compiler automatically searches for any applicable implicit conversions to types that provide the method in question.
This technique allows new methods to be added to an existing class using an add-on library such that only code that imports the add-on library gets the new functionality, and all other code is unaffected.
The following example shows the enrichment of type Int
with methods isEven
and isOdd
:
object MyExtensions:
extension (i: Int)
def isEven = i % 2 == 0
def isOdd = !isEven
import MyExtensions._ // bring implicit enrichment into scope
4.isEven // -> true
Importing the members of MyExtensions
brings the implicit conversion to extension class IntPredicates
into scope.[43]
Concurrency
Scala's standard library includes support for futures and promises, in addition to the standard Java concurrency APIs. Originally, it also included support for the actor model, which is now available as a separate source-available platform Akka[44] licensed by Lightbend Inc. Akka actors may be distributed or combined with software transactional memory (transactors). Alternative communicating sequential processes (CSP) implementations for channel-based message passing are Communicating Scala Objects,[45] or simply via JCSP.
An Actor is like a thread instance with a mailbox. It can be created by system.actorOf
, overriding the receive
method to receive messages and using the !
(exclamation point) method to send a message.[46]
The following example shows an EchoServer that can receive messages and then print them.
val echoServer = actor(new Act:
become:
case msg => println("echo " + msg)
)
echoServer ! "hi"
Scala also comes with built-in support for data-parallel programming in the form of Parallel Collections[47] integrated into its Standard Library since version 2.9.0.
The following example shows how to use Parallel Collections to improve performance.[48]
val urls = List("https://scala-lang.org", "https://github.com/scala/scala")
def fromURL(url: String) = scala.io.Source.fromURL(url)
.getLines().mkString("\n")
val t = System.currentTimeMillis()
urls.par.map(fromURL(_)) // par returns parallel implementation of a collection
println("time: " + (System.currentTimeMillis - t) + "ms")
Besides futures and promises, actor support, and data parallelism, Scala also supports asynchronous programming with software transactional memory, and event streams.[49]
Cluster computing
The most well-known open-source cluster-computing solution written in Scala is Apache Spark. Additionally, Apache Kafka, the publish–subscribe message queue popular with Spark and other stream processing technologies, is written in Scala.
Testing
There are several ways to test code in Scala. ScalaTest supports multiple testing styles and can integrate with Java-based testing frameworks.[50] ScalaCheck is a library similar to Haskell's QuickCheck.[51] specs2 is a library for writing executable software specifications.[52] ScalaMock provides support for testing high-order and curried functions.[53] JUnit and TestNG are popular testing frameworks written in Java.
Versions
Comparison with other JVM languages
Scala is often compared with Groovy and Clojure, two other programming languages also using the JVM. Substantial differences between these languages exist in the type system, in the extent to which each language supports object-oriented and functional programming, and in the similarity of their syntax to that of Java.
Scala is statically typed, while both Groovy and Clojure are dynamically typed. This makes the type system more complex and difficult to understand but allows almost all[39] type errors to be caught at compile-time and can result in significantly faster execution. By contrast, dynamic typing requires more testing to ensure program correctness, and thus is generally slower, to allow greater programming flexibility and simplicity. Regarding speed differences, current versions of Groovy and Clojure allow optional type annotations to help programs avoid the overhead of dynamic typing in cases where types are practically static. This overhead is further reduced when using recent versions of the JVM, which has been enhanced with an invoke dynamic instruction for methods that are defined with dynamically typed arguments. These advances reduce the speed gap between static and dynamic typing, although a statically typed language, like Scala, is still the preferred choice when execution efficiency is very important.
Regarding programming paradigms, Scala inherits the object-oriented model of Java and extends it in various ways. Groovy, while also strongly object-oriented, is more focused in reducing verbosity. In Clojure, object-oriented programming is deemphasised with functional programming being the main strength of the language. Scala also has many functional programming facilities, including features found in advanced functional languages like Haskell, and tries to be agnostic between the two paradigms, letting the developer choose between the two paradigms or, more frequently, some combination thereof.
Regarding syntax similarity with Java, Scala inherits much of Java's syntax, as is the case with Groovy. Clojure on the other hand follows the Lisp syntax, which is different in both appearance and philosophy.[citation needed]
Adoption
Language rankings
Back in 2013, when Scala was in version 2.10, the ThoughtWorks Technology Radar, which is an opinion based biannual report of a group of senior technologists,[123] recommended Scala adoption in its languages and frameworks category.[124]
In July 2014, this assessment was made more specific and now refers to a “Scala, the good parts”, which is described as “To successfully use Scala, you need to research the language and have a very strong opinion on which parts are right for you, creating your own definition of Scala, the good parts.”.[125]
In the 2018 edition of the State of Java survey,[126] which collected data from 5160 developers on various Java-related topics, Scala places third in terms of use of alternative languages on the JVM. Relative to the prior year's edition of the survey, Scala's use among alternative JVM languages fell from 28.4% to 21.5%, overtaken by Kotlin, which rose from 11.4% in 2017 to 28.8% in 2018. The Popularity of Programming Language Index,[127] which tracks searches for language tutorials, ranked Scala 15th in April 2018 with a small downward trend, and 17th in Jan 2021. This makes Scala the 3rd most popular JVM-based language after Java and Kotlin, ranked 12th.
The RedMonk Programming Language Rankings, which establishes rankings based on the number of GitHub projects and questions asked on Stack Overflow, in January 2021 ranked Scala 14th.[128] Here, Scala was placed inside a second-tier group of languages–ahead of Go, PowerShell, and Haskell, and behind Swift, Objective-C, Typescript, and R.
The TIOBE index[129] of programming language popularity employs internet search engine rankings and similar publication-counting to determine language popularity. In September 2021, it showed Scala in 31st place. In this ranking, Scala was ahead of Haskell (38th) and Erlang, but below Go (14th), Swift (15th), and Perl (19th).
As of 2022, JVM-based languages such as Clojure, Groovy, and Scala are highly ranked, but still significantly less popular than the original Java language, which is usually ranked in the top three places.[128][129]
Companies
- In April 2009, Twitter announced that it had switched large portions of its backend from Ruby to Scala and intended to convert the rest.[130]
- Tesla, Inc. uses Akka with Scala in the backend of the Tesla Virtual Power Plant. Thereby, the Actor model is used for representing and operating devices that together with other components make up an instance of the virtual power plant, and Reactive Streams are used for data collection and data processing.[131]
- Apache Kafka is implemented in Scala with regards to most of its core and other critical parts. It is maintained and extended through the open source project and by the company Confluent.[132]
- Gilt uses Scala and Play Framework.[133]
- Foursquare uses Scala and Lift.[134]
- Coursera uses Scala and Play Framework.[135]
- Apple Inc. uses Scala in certain teams, along with Java and the Play framework.[136][137]
- The Guardian newspaper's high-traffic website guardian.co.uk[138] announced in April 2011 that it was switching from Java to Scala.[139][140]
- The New York Times revealed in 2014 that its internal content management system Blackbeard is built using Scala, Akka, and Play.[141]
- The Huffington Post newspaper started to employ Scala as part of its content delivery system Athena in 2013.[142]
- Swiss bank UBS approved Scala for general production use.[143]
- LinkedIn uses the Scalatra microframework to power its Signal API.[144]
- Meetup uses Unfiltered toolkit for real-time APIs.[145]
- Remember the Milk uses Unfiltered toolkit, Scala and Akka for public API and real-time updates.[146]
- Verizon seeking to make "a next-generation framework" using Scala.[147]
- Airbnb develops open-source machine-learning software "Aerosolve", written in Java and Scala.[148]
- Zalando moved its technology stack from Java to Scala and Play.[149]
- SoundCloud uses Scala for its back-end, employing technologies such as Finagle (micro services),[150] Scalding and Spark (data processing).[151]
- Databricks uses Scala for the Apache Spark Big Data platform.
- Morgan Stanley uses Scala extensively in their finance and asset-related projects.[152]
- There are teams within Google and Alphabet Inc. that use Scala, mostly due to acquisitions such as Firebase[153] and Nest.[154]
- Walmart Canada uses Scala for their back-end platform.[155]
- Duolingo uses Scala for their back-end module that generates lessons.[156]
- HMRC uses Scala for many UK Government tax applications.[157]
- M1 Finance uses Scala for their back-end platform.[158]
Criticism
In November 2011, Yammer moved away from Scala for reasons that included the learning curve for new team members and incompatibility from one version of the Scala compiler to the next.[159] In March 2015, former VP of the Platform Engineering group at Twitter Raffi Krikorian, stated that he would not have chosen Scala in 2011 due to its learning curve.[160] The same month, LinkedIn SVP Kevin Scott stated their decision to "minimize [their] dependence on Scala".[161]
See also
- sbt, a widely used build tool for Scala projects
- Spark Framework is designed to handle, and process big-data and it solely supports Scala
- Neo4j is a java spring framework supported by Scala with domain-specific functionality, analytical capabilities, graph algorithms, and many more
- Play!, an open-source Web application framework that supports Scala
- Akka, an open-source toolkit for building concurrent and distributed applications
- Chisel, an open-source language built on Scala that is used for hardware design and generation.[162]
References
- ^ "Scala 3.4.0".
- ^ "Notice file". GitHub. 2019-01-24. Retrieved 2019-12-04.
- ^ "Scala Macros".
- ^ Fogus, Michael (6 August 2010). "MartinOdersky take(5) toList". Send More Paramedics. Retrieved 2012-02-09.
- ^ a b c d Odersky, Martin (11 January 2006). "The Scala Experiment - Can We Provide Better Language Support for Component Systems?" (PDF). Retrieved 2016-06-22.
- ^ a b c d Odersky, Martin; et al. (2006). "An Overview of the Scala Programming Language" (PDF) (2nd ed.). École Polytechnique Fédérale de Lausanne (EPFL). Archived (PDF) from the original on 2020-07-09.
- ^ Odersky, Martin (2008). Programming in Scala. Mountain View, California: Artima. p. 3. ISBN 9780981531601. Retrieved 12 June 2014.
- ^ Wampler, Dean; Payne, Alex (15 September 2009). Programming Scala: Scalability = Functional Programming + Objects. O'Reilly Media, Inc. p. 7. ISBN 978-1-4493-7926-1. Retrieved 13 May 2024.
The creators of Scala actually pronounce it scah-lah, like the Italian word for "stairs." The two "a"s are pronounced the same.
- ^ Potvin, Pascal; Bonja, Mario (24 September 2015). SDL 2013: Model-Driven Dependability Engineering. Lecture Notes in Computer Science. Vol. 7916. arXiv:1509.07326. doi:10.1007/978-3-642-38911-5. ISBN 978-3-642-38910-8. S2CID 1214469.
- ^ "Frequently Asked Questions: Java Interoperability". Scala-lang.org. Retrieved 2015-02-06.
- ^ Martin Odersky (17 June 2020). Martin Odersky: A Scala 3 Update (video). YouTube. Event occurs at 36:35–45:08. Archived from the original on 2021-12-21. Retrieved 2021-04-24.
- ^ "Effect expt". scala. Retrieved 2022-07-31.
- ^ Loverdo, Christos (2010). Steps in Scala: An Introduction to Object-Functional Programming. Cambridge University Press. p. xiii. ISBN 9781139490948. Retrieved 31 July 2014.
- ^ a b c d Odersky, Martin (9 June 2006). "A Brief History of Scala". Artima.com.
- ^ a b c d Odersky, M.; Rompf, T. (2014). "Unifying functional and object-oriented programming with Scala". Communications of the ACM. 57 (4): 76. doi:10.1145/2591013.
- ^ Martin Odersky, "The Scala Language Specification Version 2.7"
- ^ "Scala Team Wins ERC Grant". Retrieved 4 July 2015.
- ^ "Commercial Support for Scala". 2011-05-12. Retrieved 2011-08-18.
- ^ "Why We Invested in Typesafe: Modern Applications Demand Modern Tools". 2011-05-12. Retrieved 2018-05-08.
- ^ "Open-source Scala gains commercial backing". 2011-05-12. Retrieved 2011-10-09.
- ^ "Cloud computing pioneer Martin Odersky takes wraps off his new company Typesafe". 2011-05-12. Retrieved 2011-08-24.
- ^ "Scala 3 Roadmap for 2024". virtuslab. Retrieved 2024-06-13.
- ^ "Scala on Android". Archived from the original on 20 June 2016. Retrieved 8 June 2016.
- ^ "Scala 2.12.8 is now available!". 2018-12-04. Retrieved 2018-12-09.
- ^ "Scala Js Is No Longer Experimental | The Scala Programming Language". Scala-lang.org. Retrieved 28 October 2015.
- ^ "Releases · scala-js/Scala-js". GitHub.
- ^ Krill, Paul (15 March 2017). "Scaled-down Scala variant cuts ties to the JVM". InfoWorld. Retrieved 21 March 2017.
- ^ Krill, Paul (2016-05-11). "Scala language moves closer to bare metal". InfoWorld.
- ^ Expunged the .net backend. by paulp · Pull Request #1718 · scala/scala · GitHub. Github.com (2012-12-05). Retrieved on 2013-11-02.
- ^ "Getting Started with Scala". Scala-lang.org. 15 July 2008. Retrieved 31 July 2014.
- ^ "Home". Blog.lostlake.org. Archived from the original on 31 August 2010. Retrieved 2013-06-25.
- ^ Scala's built-in control structures such as
if
orwhile
cannot be re-implemented. There is a research project, Scala-Virtualized, that aimed at removing these restrictions: Adriaan Moors, Tiark Rompf, Philipp Haller and Martin Odersky. Scala-Virtualized. Proceedings of the ACM SIGPLAN 2012 workshop on Partial evaluation and program manipulation, 117–120. July 2012. - ^ a b "Pimp my Library". Artima.com. 2006-10-09. Retrieved 2013-06-25.
- ^ "Expressions | Scala 2.13". scala-lang.org. Retrieved 2021-05-24.
- ^ "Mutable and Immutable Collections - Scala Documentation". Retrieved 30 April 2020.
- ^ "Collections - Concrete Immutable Collection Classes - Scala Documentation". Retrieved 4 July 2015.
- ^ Dougherty, Rich. "Rich Dougherty's blog". Retrieved 4 July 2015.
- ^ "TailCalls - Scala Standard Library API (Scaladoc) 2.10.2 - scala.util.control.TailCalls". Scala-lang.org. Retrieved 2013-06-25.
- ^ a b "Java and Scala's Type Systems are Unsound" (PDF).
- ^ Giarrusso, Paolo G. (2013). "Reify your collection queries for modularity and speed!". Proceedings of the 12th annual international conference on Aspect-oriented software development. ACM. arXiv:1210.6284. Bibcode:2012arXiv1210.6284G.
Also known as pimp-my-library pattern
- ^ Gilbert, Clint (2011-11-15). "What is highest priority for Scala to succeed in corporate world (Should be in scala-debate?) ?". Scala-lang.org. Retrieved 2019-05-08.
- ^ "Should we "enrich" or "pimp" Scala libraries?". stackexchange.com. 17 June 2013. Retrieved 15 April 2016.
- ^ Implicit classes were introduced in Scala 2.10 to make method extensions more concise. This is equivalent to adding a method
implicit def IntPredicate(i: Int) = new IntPredicate(i)
. The class can also be defined asimplicit class IntPredicates(val i: Int) extends AnyVal { ... }
, producing a so-called value class, also introduced in Scala 2.10. The compiler will then eliminate actual instantiations and generate static methods instead, allowing extension methods to have virtually no performance overhead. - ^ What is Akka?, Akka online documentation
- ^ Sufrin, Bernard (2008). "Communicating Scala Objects". In Welch, P. H.; Stepney, S.; Polack, F.A.C.; Barnes, F. R. M.; McEwan, A.A.; Stiles, G.S.; Broenink, J. F.; Sampson, A. T. (eds.). Communicating Process Architectures 2008: WoTUG-31 (PDF). IOS Press. ISBN 978-1586039073.
- ^ Yan, Kay. "Scala Tour". Retrieved 4 July 2015.
- ^ "Parallelcollections - Overview - Scala Documentation". Docs.scala-lang.org. Retrieved 2013-06-25.
- ^ Yan, Kay. "Scala Tour". Retrieved 4 July 2015.
- ^ Learning Concurrent Programming in Scala, Aleksandar Prokopec, Packt Publishing
- ^ Kops, Micha (2013-01-13). "A short Introduction to ScalaTest". hascode.com. Retrieved 2014-11-07.
- ^ Nilsson, Rickard (2008-11-17). "ScalaCheck 1.5". Scala-lang.org. Retrieved 2014-11-07.
- ^ "Build web applications using Scala and the Play Framework". workwithplay.com. 2013-05-22. Retrieved 2014-11-07.
- ^ Butcher, Paul (2012-06-04). "ScalaMock 3.0 Preview Release". paulbutcher.com. Archived from the original on 2014-11-08. Retrieved 2014-11-07.
- ^ a b c d e f g "Scala Change History". Scala-lang.org. Archived from the original on 2007-10-09.
- ^ "Dropped: XML Literals". dotty.epfl.ch. Retrieved 2021-03-05.
- ^ "Changes in Version 2.0 (12-Mar-2006)". Scala-lang.org. 2006-03-12. Retrieved 2014-11-07.
- ^ "Changes in Version 2.1.8 (23-Aug-2006)". Scala-lang.org. 2006-08-23. Retrieved 2014-11-07.
- ^ "Changes in Version 2.3.0 (23-Nov-2006)". Scala-lang.org. 2006-11-23. Retrieved 2014-11-07.
- ^ "Changes in Version 2.4.0 (09-Mar-2007)". Scala-lang.org. 2007-03-09. Retrieved 2014-11-07.
- ^ "Changes in Version 2.5 (02-May-2007)". Scala-lang.org. 2007-05-02. Retrieved 2014-11-07.
- ^ "Changes in Version 2.6 (27-Jul-2007)". Scala-lang.org. 2007-06-27. Retrieved 2014-11-07.
- ^ "Changes in Version 2.7.0 (07-Feb-2008)". Scala-lang.org. 2008-02-07. Retrieved 2014-11-07.
- ^ "Changes in Version 2.8.0 (14-Jul-2010)". Scala-lang.org. 2010-07-10. Retrieved 2014-11-07.
- ^ "Changes in Version 2.9.0 (12-May-2011)". Scala-lang.org. 2011-05-12. Retrieved 2014-11-07.
- ^ "Changes in Version 2.10.0". Scala-lang.org. 2013-01-04. Retrieved 2014-11-07.
- ^ Harrah, Mark. "Value Classes and Universal Traits". Scala-lang.org. Retrieved 2014-11-07.
- ^ Suereth, Josh. "SIP-13 - Implicit classes". Scala-lang.org. Archived from the original on 2014-11-08. Retrieved 2014-11-07.
- ^ Suereth, Josh. "String Interpolation". Scala-lang.org. Retrieved 2014-11-07.
- ^ Haller, Philipp; Prokopec, Aleksandar. "Futures and Promises". Scala-lang.org. Retrieved 2014-11-07.
- ^ "SIP-17 - Type Dynamic". Scala-lang.org. Archived from the original on 2014-11-08. Retrieved 2014-11-07.
- ^ "SIP-18 - Modularizing Language Features". Scala-lang.org. Archived from the original on 2014-11-08. Retrieved 2014-11-07.
- ^ Prokopec, Aleksandar; Miller, Heather. "Parallel Collections". Scala-lang.org. Retrieved 2014-11-07.
- ^ Miller, Heather; Burmako, Eugene. "Reflection Overview". Scala-lang.org. Retrieved 2014-11-07.
- ^ Burmako, Eugene. "Def Macros". Scala-lang.org. Retrieved 2014-11-07.
- ^ "Scala 2.10.2 is now available!". Scala-lang.org. 2013-06-06. Archived from the original on 2014-11-08. Retrieved 2014-11-07.
- ^ "Scala 2.10.3 is now available!". Scala-lang.org. 2013-10-01. Archived from the original on 2014-11-08. Retrieved 2014-11-07.
- ^ "Scala 2.10.4 is now available!". Scala-lang.org. 2014-03-18. Retrieved 2015-01-07.
- ^ "Scala 2.10.5 is now available!". Scala-lang.org. 2015-03-04. Retrieved 2015-03-23.
- ^ "Scala 2.11.0 is now available!". Scala-lang.org. 2014-04-21. Retrieved 2014-11-07.
- ^ "Scala 2.11.1 is now available!". Scala-lang.org. 2014-05-20. Retrieved 2014-11-07.
- ^ "Scala 2.11.2 is now available!". Scala-lang.org. 2014-07-22. Retrieved 2014-11-07.
- ^ "Scala 2.11.4 is now available!". Scala-lang.org. 2014-10-30. Retrieved 2014-11-07.
- ^ "Scala 2.11.5 is now available!". Scala-lang.org. 2015-01-08. Retrieved 2015-01-22.
- ^ "Scala 2.11.6 is now available!". Scala-lang.org. 2015-03-05. Retrieved 2015-03-12.
- ^ "Scala 2.11.7 is now available!". Scala-lang.org. 2015-06-23. Retrieved 2015-07-03.
- ^ "Scala 2.11.8 is now available!". Scala-lang.org. 2016-03-08. Retrieved 2016-03-09.
- ^ a b "Three new releases and more GitHub goodness!". Scala-lang.org. 2017-04-18. Retrieved 2017-04-19.
- ^ "Security update: 2.12.4, 2.11.12, 2.10.7 (CVE-2017-15288)". Scala-lang.org. 2017-11-13. Retrieved 2018-05-04.
- ^ "Scala 2.12.0 is now available!". Scala-lang.org. 2016-11-03. Retrieved 2017-01-08.
- ^ "Scala 2.12.1 is now available!". Scala-lang.org. 2016-12-05. Retrieved 2017-01-08.
- ^ "Scala 2.12.3 is now available!". Scala-lang.org. 2017-07-26. Retrieved 2017-08-16.
- ^ "Scala 2.12.4 is now available!". Scala-lang.org. 2017-10-18. Retrieved 2017-10-26.
- ^ "Scala 2.12.5 is now available!". Scala-lang.org. 2018-03-15. Retrieved 2018-03-20.
- ^ "Scala 2.12.6 is now available!". Scala-lang.org. 2018-04-27. Retrieved 2018-05-04.
- ^ "Scala 2.12.7 is now available!". Scala-lang.org. 2018-09-27. Retrieved 2018-10-09.
- ^ "Scala 2.12.8 is now available!". Scala-lang.org. 2018-12-04. Retrieved 2018-12-09.
- ^ "Scala 2.12.9 is now available!". Scala-lang.org. 2019-08-05. Retrieved 2021-01-20.
- ^ "Scala 2.12.10 is now available!". Scala-lang.org. 2019-09-10. Retrieved 2021-01-20.
- ^ "Scala 2.12.11 is now available!". Scala-lang.org. 2020-03-16. Retrieved 2021-01-20.
- ^ "Scala 2.12.12 is now available!". Scala-lang.org. 2020-07-13. Retrieved 2021-01-20.
- ^ "Scala 2.12.13 is now available!". Scala-lang.org. 2021-01-12. Retrieved 2021-01-20.
- ^ "Scala 2.12.14 is now available!". Scala-lang.org. 2021-05-28. Retrieved 2022-04-15.
- ^ "Scala 2.12.15 is now available!". Scala-lang.org. 2021-09-14. Retrieved 2022-06-19.
- ^ "Scala 2.12.16 is now available!". Scala-lang.org. 2022-06-10. Retrieved 2022-06-19.
- ^ "Scala 2.12.17 is now available!". Scala-lang.org. 2022-06-10. Retrieved 2022-09-16.
- ^ "Scala 2.12.18 is now available!". Scala-lang.org. 2022-06-10. Retrieved 2023-06-07.
- ^ "Scala 2.13.0 is now available!". Scala-lang.org. 2019-06-11. Retrieved 2018-06-17.
- ^ "Scala 2.13.1 is now available!". Scala-lang.org. 2019-09-18. Retrieved 2021-01-20.
- ^ "Scala 2.13.2 is now available!". Scala-lang.org. 2020-04-22. Retrieved 2021-01-20.
- ^ "Scala 2.13.3 is now available!". Scala-lang.org. 2020-06-25. Retrieved 2021-01-20.
- ^ "Scala 2.13.4 is now available!". Scala-lang.org. 2020-11-19. Retrieved 2021-01-20.
- ^ "Scala 2.13.5 is now available!". Scala-lang.org. 2021-02-22. Retrieved 2021-02-26.
- ^ "Scala 2.13.6 is now available!". Scala-lang.org. 2021-05-17. Retrieved 2022-04-15.
- ^ "Scala 2.13.7 is now available!". Scala-lang.org. 2021-11-01. Retrieved 2022-04-15.
- ^ "Scala 2.13.8 is now available!". Scala-lang.org. 2022-01-12. Retrieved 2022-04-15.
- ^ "Scala 2.13.9 is now available!". Scala-lang.org. 2022-09-21. Retrieved 2023-08-28.
- ^ "Scala 2.13.10 is now available!". Scala-lang.org. 2022-10-13. Retrieved 2023-08-28.
- ^ "Scala 2.13.11 is now available!". Scala-lang.org. 2023-06-07. Retrieved 2023-08-28.
- ^ "Scala 3 is here!". Scala-lang.org. 2021-05-14. Retrieved 2021-05-26.
- ^ "Scala 3.1.2". Scala-lang.org. 2022-04-12. Retrieved 2022-06-19.
- ^ "Scala 3.2.2". Scala-lang.org. 2023-01-30. Retrieved 2023-08-28.
- ^ "Scala 3.3.0". Scala-lang.org. 2023-05-30. Retrieved 2023-08-28.
- ^ "ThoughtWorks Technology Radar FAQ".
- ^ "ThoughtWorks Technology Radar MAY 2013" (PDF).
- ^ "Scala, the good parts".
- ^ "The State of Java in 2018".
- ^ "Popularity of Programming Language Index".
- ^ a b O'Grady, Stephen (1 March 2021). "The RedMonk Programming Language Rankings: January 2021". RedMonk.
- ^ a b "TIOBE Index for May 2021".
- ^ Greene, Kate (1 April 2009). "The Secret Behind Twitter's Growth, How a new Web programming language is helping the company handle its increasing popularity". Technology Review. MIT. Retrieved 6 April 2009.
- ^ Breck, Colin; Link, Percy (2020-03-23). "Tesla Virtual Power Plant (Architecture and Design)". Retrieved 2023-03-28.
- ^ "Apache Kafka source code at GitHub". Apache Software Foundation. Retrieved 29 March 2023.
- ^ "Play Framework, Akka and Scala at Gilt Groupe". Lightbend. 15 July 2013. Retrieved 16 July 2016.
- ^ "Scala, Lift, and the Future". Archived from the original on 13 January 2016. Retrieved 4 July 2015.
- ^ Saeta, Brennan (2014-02-17). "Why we love Scala at Coursera". Coursera Engineering. Retrieved 2023-09-21.
- ^ "Apple Engineering PM Jarrod Nettles on Twitter". Jarrod Nettles. Retrieved 2016-03-11.
- ^ "30 Scala job openings at Apple". Alvin Alexander. Retrieved 2016-03-11.
- ^ David Reid & Tania Teixeira (26 February 2010). "Are people ready to pay for online news?". BBC. Retrieved 2010-02-28.
- ^ "Guardian switching from Java to Scala". Heise Online. 2011-04-05. Retrieved 2011-04-05.
- ^ "Guardian.co.uk Switching from Java to Scala". InfoQ.com. 2011-04-04. Retrieved 2011-04-05.
- ^ Roy, Suman; Sundaresan, Krishna (2014-05-13). "Building Blackbeard: A Syndication System Powered By Play, Scala and Akka". The New York Times. Retrieved 2014-07-20.
- ^ Pavley, John (2013-08-11). "Sneak Peek: HuffPost Brings Real Time Collaboration to the Newsroom". Huffington Post. Retrieved 2014-07-20.
- ^ Binstock, Andrew (2011-07-14). "Interview with Scala's Martin Odersky". Dr. Dobb's Journal. Retrieved 2012-02-10.
- ^ Synodinos, Dionysios G. (2010-10-11). "LinkedIn Signal: A Case Study for Scala, JRuby and Voldemort". InfoQ.
- ^ "Real-life Meetups Deserve Real-time APIs".
- ^ "Real time updating comes to the Remember The Milk web app".
- ^ "WHAT IS SCALA". 8 March 2023. Retrieved 2023-03-17.
- ^ Novet, Jordan (2015-06-04). "Airbnb announces Aerosolve, an open-source machine learning software package". Retrieved 2016-03-09.
- ^ Kops, Alexander (2015-12-14). "Zalando Tech: From Java to Scala in Less Than Three Months". Retrieved 2016-03-09.
- ^ Calçado, Phil (2014-06-13). "Building Products at SoundCloud—Part III: Microservices in Scala and Finagle". Retrieved 2016-03-09.
- ^ "Customer Case Studies: SoundCloud". Concurrent Inc. 2014-11-18. Retrieved 2016-03-09.
- ^ Scala at Morgan Stanley (video). Skills Matter. 2015-12-03. Retrieved 2016-03-11.
- ^ Greg Soltis (2015-12-03). SF Scala, Greg Soltis: High Performance Services in Scala (video). Skills Matter. Archived from the original on 2021-12-21. Retrieved 2016-03-11.
- ^ Lee Mighdoll. "Scala jobs at Nest". Retrieved 2016-03-11.
- ^ Nurun. "Nurun Launches Redesigned Transactional Platform With Walmart Canada". Retrieved 2013-12-11.
- ^ Horie, André K. (2017-01-31). "Rewriting Duolingo's engine in Scala". Retrieved 2017-02-03.
- ^ "HMRC GitHub repository". GitHub.
- ^ "Meet M1 Finance, A ScalaCon Gold Sponsor". ScalaCon. Retrieved 2023-09-02.
- ^ Hale, Coda (29 November 2011). "The Rest of the Story". codahale.com. Retrieved 7 November 2013.
- ^ Krikorian, Raffi (17 March 2015). O'Reilly Software Architecture Conference 2015 Complete Video Compilation: Re-Architecting on the Fly - Raffi Krikorian - Part 3 (video). O'Reilly Media. Event occurs at 4:57. Retrieved 8 March 2016.
What I would have done differently four years ago is use Java and not used Scala as part of this rewrite. [...] it would take an engineer two months before they're fully productive and writing Scala code.
[permanent dead link] - ^ Scott, Kevin (11 Mar 2015). "Is LinkedIn getting rid of Scala?". quora.com. Retrieved 25 January 2016.
- ^ "Chisel: Constructing Hardware in a Scala Embedded Language". UC Berkeley APSIRE. Retrieved 27 May 2020.
Further reading
- Odersky, Martin; Spoon, Lex; Venners, Bill (15 December 2019). Programming in Scala: A Comprehensive Step-by-step Guide (4th ed.). Artima Inc. p. 896. ISBN 978-0-9815316-1-8.
- Horstmann, Cay (15 December 2016). Scala for the Impatient (2nd ed.). Addison-Wesley Professional. p. 384. ISBN 978-0-134-54056-6.
- Wampler, Dean; Payne, Alex (14 December 2014). Programming Scala: Scalability = Functional Programming + Objects (2nd ed.). O'Reilly Media. p. 583. ISBN 978-1-491-94985-6.
- Suereth, Joshua D. (Spring 2011). Scala in Depth. Manning Publications. p. 225. ISBN 978-1-935182-70-2.
- Meredith, Gregory (2011). Monadic Design Patterns for the Web (PDF) (1st ed.). p. 300.
- Odersky, Martin; Spoon, Lex; Venners, Bill (10 December 2008). Programming in Scala, eBook (1st ed.). Artima Inc.